Matlab 13 Codes for Simulation and Modeling - All Simulation Code
Matlab 13 Codes for Simulation and Modeling
There are most important Matlab codes for Simulation and Modeling Course. These codes are done by me and with some of my friends. Error's are not impossible. See these codes for Simulation and modeling course.
[Having any error, please comment]
- Mid Square Random Number Generator Matlab code
- Residue Method / Mixed Multiplicative Random Number Generator Matlab code
- Additive Congruential Random Number Generator Matlab code
- Multiplicative Congruential Random Number Generator Matlab code
- Arithmetic congruential Method Random Number Generator Matlab code
- Binomial Distribution Matlab code
- Poison Distribution Matlab code
- Chi Square Test for a given set of random numbers
- Monte Carlo - Pure Pursuit Code For Matlab
- Monte Carlo - Gambling Game Code For Matlab
- Monte Carlo - Monte Carlo Integration Code For Matlab
- Monte Carlo - Monte Carlo Pi Code For Matlab
- Single Server Queuing System Matlab Code
Mid Square Method Random Number Generator Code
totalNumber = input('Number of Random Numbers want to generate : ');
choose = input('Enter the seed : ');
disp('Total Random Numbers are : ');
for i = 1:totalNumber
random = choose ^ 2;
random = random / 100; % Find the dividend
random = rem(random, 10000); % Get the reminder;
choose = random;
fprintf('%.2f ', random);
end
fprintf('\n');
Output Mid square random Number generator:
Residue Method / Mixed Multiplicative Random Number Generator Code
clc;
a=input('Please Enter the value of a : ');
b=input('Please Enter the value of b : ');
m=input('Please Enter the value of m : ');
r=input('Please Enter the value of seed or r : ');
totalNumber = input('Number of Random Numbers want to generate : ');
for i = 1:totalNumber
r = mod(a*r +b, m);
fprintf('%4.0f ', r);
end
fprintf('\n');
Output Residue Method random Number generator:
Additive Congruential Method Random Number Generator Code
Same as residue method in this case just a = 1 and hence rules changed
%% r(i+1) = (r(i) + b) mod m
clc;
b=input('Please Enter the value of b : ');
m=input('Please Enter the value of m : ');
r=input('Please Enter the value of seed or r : ');
totalNumber = input('Number of Random Numbers want to generate : ');
for i = 1:totalNumber
r = mod(r +b, m);
fprintf('%4.0f ', r);
end
fprintf('\n');
Output Additive Congruential Method random Number generator:
Multiplicative Congruential Method Random Number Generator Code
Same as residue method in this case just b = 0 and hence rules changed
%% r(i+1) = (ar(i)) mod m
clc;
a=input('Please Enter the value of a : ');
m=input('Please Enter the value of m : ');
r=input('Please Enter the value of seed or r : ');
totalNumber = input('Number of Random Numbers want to generate : ');
for i = 1:totalNumber
r = mod(a*r, m);
fprintf('%4.0f ', r);
end
fprintf('\n');
Output Multiplicative Congruential Method random Number generator:
Arithmetic congruential Random Number Generator Code
%% r(i + 1) = (r(i) + r(i - 1)) percent m
clc;
N = input('Enter How Many Number You want to generate = ');
r0 = input('Enter First Random Number = ');
r1 = input('Enter Second Random Number = ');
m = input('Enter Maximum Range Of Random Number = ');
for i = 1:N
r2 = r0 + r1;
random = mod(r2, m);
fprintf('%d ', random);
r0 = r1;
r1 = r2;
end
Output Arithmetic congruential random Number generator:
Binomial Distribution Code
clc;
trial = input ('Type the number of trial = ');
p = input('Type the value of p(<1.0) the probability of success = ');
variate = input('Type the number of variates to be generated = ');
fprintf('Here,You gave \t Number of trial = %d, Probability of success = %d\nNumber of variates = %d\n',trial,p,variate);
x = 0; n = trial;
for i = 1:n
y = rand();
if(y<p)
x = x+1;
end
fprintf('x = %d\n', x);
end
Output Binomial Distribution :
Poison Distribution Code
clc;
lamda_value = input('Value of Lamda = ');
number_values = input('Number Of Values = ');
for i=1:number_values
count = 0;
p = 0;
fact = 1;
y = rand;
while(y > p)
prob = (power(lamda_value,count)*power(2.718282,-lamda_value))/fact; %Use the poison rules
p = p + prob;
count = count + 1;
fact = fact * count;
end
fprintf('%.f\t',count);
fprintf('\n');
end
fprintf('\n');
Output Poison Distribution :
Chi Square Test for a given set of random numbers Matlab Code
clc;
r = [36 91 51 02 54 06 58 06 58 02 54 01 48 97 43 22 83 25 79 95 42 87 73 17 02 42 95 38 79 29 65 09 55 97 39 83 31 77 17 67 62 03 49 90 37 13 17 58 11 51 92 33 78 21 66 09 54 49 90 35 84 26 74 22 62 12 90 36 83 32 75 31 94 34 87 40 07 58 05 56 22 58 77 71 10 73 23 57 13 36 89 22 68 02 44 99 27 81 26 85 ];
r_length = length(r);
fprintf('Total Students = %d\n', r_length);
for i = 1:r_length
fprintf(' %d ', r(i));
end
fprintf('\n');
Acceptance_value = input('Enter acceptance value at that confidence level = ');
Expected = input('Enter Expected value = ');
E = Expected;
Range = Expected * Expected;
frequency = 0;
diff_square = 0;
i = 0;
while i < Range
for j = 1:r_length
if((r(j) > i) && (r(j) <= Expected))
frequency = frequency + 1;
end
end
O = frequency;
diff_square = diff_square + (abs(O - E)) ^ 2;
fprintf('Difference Square = %d\n', frequency);
i = i + E;
Expected = Expected + E;
frequency = 0;
end
chi_square = double(diff_square / E);
fprintf('\n\nChi Square value is = %f\n', chi_square);
if (Acceptance_value > chi_square)
fprintf('\nThe random numbers follows the chi-square test. Because Chi Square value %.2f is less than Acceptance value %.2f\n', chi_square, Acceptance_value);
else
fprintf('\nThe random numbers follows the chi-square test. Because Chi Square value %.2f is greater than Acceptance value %.2f\n', chi_square, Acceptance_value);
end
fprintf('\n\n');
Output Chi Square Test Code :
Monte Carlo - Pure pursuit Bombers and Fighters Matlab Code
clc;
hold all;
xb=[100 110 120 129 140 149 158 168 179 188 198 209 219 226 234 240];
yb=[0 3 6 10 15 20 26 32 37 34 30 27 23 19 16 14];
xf = [];
yf = [];
xf(1)=0;
yf(1)=50;
s=20;
dist=0;
for i=1:15
pause on;
plot(xb(i),yb(i),'r*');
title('Pure Pursuit Problem');
pause(1);
plot(xf(i),yf(i),'g*');
y=yb(i)-yf(i);
x=xb(i)-xf(i);
dist=sqrt(y^2+x^2);
if(dist<=12)
fprintf('Bomber destroyed at %d s',i);
break;
end
xf(i+1)=xf(i)+s*((xb(i)-xf(i))/dist);
yf(i+1)=yf(i)+s*((yb(i)-yf(i))/dist);
end
Output Monte Carlo - Pure Pursuit Bombers and fighters Code :
Bomber destroyed at 11 s>>
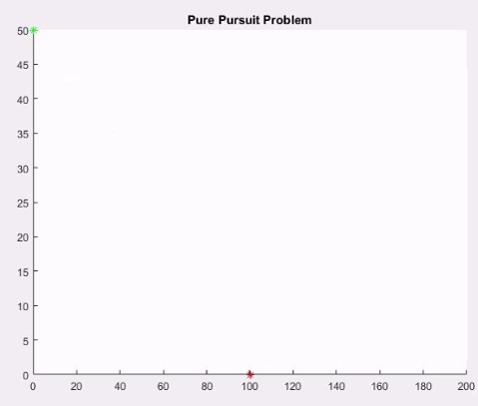
Gambling Game - Monte Carlo Matlab Code
clc;
n = input('How many time you want to play the game : ');
difference = 0;
tail = 0;
head = 0;
fprintf('\n---------------------------------------------------------------\n');
fprintf('Game No.\tSl No.\tRandom Number\tHeads\tTails\tDifference');
fprintf('\n---------------------------------------------------------------\n');
for i = 1:n
j = 1;
while 1
toss = int8((10-0)*rand(1) + 0); %Generate a random number between 0 to 10%
if (toss > 4)
tail = tail + 1;
else
head = head + 1;
end
difference = abs((head - tail));
fprintf('\n\t%d\t\t%d\t\t\t%d\t\t\t%d\t\t%d\t\t%d\t\t%d', i, j, toss, head, tail, difference);
if(difference == 3) %The rules of the Gambling - If difference = 3 then Count the lose or won coins
result = 8 - j;
if(result < 0)
fprintf('\n\t\tYou lose %d coins\n', abs(result));
else
fprintf('\n\t\tYou won %d coins\n', abs(result));
end
break;
end
j = j + 1;
end % End while
end % End For
Output Gambling Game - Monte Carlo Matlab Code :
Monte Carlo Integration - Monte Carlo Matlab Code
clc;
max_dot = input('How many times do you want to put dot : ');
inside = 0;
a = input('Enter a : ');
b = input('Enter b : ');
h = input('Enter h : ');
%Draw Plot
for x = 1: .01:10
y = x^3;
plot(x,y, 'y.');
hold on;
end
for i = 1:max_dot
x = a + rand * (b-a);
y = rand * h;
if y <= x^3
inside = inside+1;
plot(x,y, 'r.');
else
plot(x,y, 'g.');
end
hold on;
end
integration = (inside/max_dot) * ((b-a) * h);
fprintf('\nIntegration is : %f\n', integration);
Output Monte Carlo Integration - Monte Carlo Matlab Code :
Monte Carlo Pi - Monte Carlo Matlab Code
clc;
maximum_dot = input('How many times want to put Dot : ');
inside = 0;
a = input('Enter a : ');
b = input('Enter b : ');
h = input('Enter h : ');
for i = 1:maximum_dot
x = rand;
y = rand;
if sqrt(x^2 + y^2) <= 1
plot(x,y,'r.');
inside = inside + 1;
else
plot(x,y,'b.');
hold on;
end
end
fprintf('Pi = %f\n', inside / maximum_dot * 4);
Output Monte Carlo Pi - Monte Carlo Matlab Code :
Code - Single Server Queuing System Matlab Code
total=0; busy=0;
%a=randi([0 8],1,8);
a=[.4 1.6 2.1 3.8 4.0 5.6 5.8 7.2];
a_length=length(a);
arr=zeros(a_length);
%d=randi([0 9],1,5);
d=[2.4 3.1 3.3 4.9 8.6];
b=union(a,d);
l=length(b);
a_t=0;d_t=0;q=0;
axis([0 b(length(b))+1 0 length(a)]);
%Queue delay Time
figure(1);
title('Queue Delay Time');
for i=1:l-1
a_m=ismember(a,b(i));
d_m=ismember(d,b(i));
if sum(a_m)>=1
a_t=a_t+1;
end
if sum(d_m)>=1
d_t=d_t+1;
end
dif=a_t-d_t;
if dif>1
rectangle('Position',[b(i) 0 b(i+1)-b(i) dif-1],'FaceColor',[1 0 0]);
arr(dif)=arr(dif)+(b(i+1)-b(i));
end
if dif>0
busy=busy+(b(i+1)-b(i));
end
end
%Server Busy Time
b_t=0;
figure(2);
title('Server Busy Time');
axis([0 b(length(b))+1 0 length(a)]);
for i=1:l-1
a_m=ismember(a,b(i));
d_m=ismember(d,b(i));
if sum(a_m)>=1
b_t=b_t+1;
end
if sum(d_m)>=1
b_t=b_t-1;
end
if b_t>0
rectangle('Position',[b(i) 0 b(i+1)-b(i) 1],'FaceColor',[0 1 0]);
end
end
for i=1:a_length
total=total+arr(i)*(i-1);
end
disp(total);
disp(total/d(length(d)));
disp(busy);
disp(busy/d(length(d)));
Output Single Server Queuing System Matlab Code
Tags:
Mid Square Random Number Generator Matlab code,Residue Method / Mixed, Multiplicative Random Number Generator Matlab code, Additive Congruential Random Number Generator Matlab code, Multiplicative Congruential Random Number Generator Matlab codeArithmetic congruential Method Random Number Generator Matlab code , Binomial Distribution Matlab code, Poison Distribution Matlab code ,Chi Square Test for a given set of random numbers, Monte Carlo - Pure Pursuit Code For Matlab, Monte Carlo - Gambling Game Code For Matlab, Monte Carlo - Monte Carlo Integration Code For Matlab, Monte Carlo - Monte Carlo Pi Code For Matlab, Single Server Queuing System Matlab Code
Subscribe to:
Post Comments
(
Atom
)
No comments :
Post a Comment